OpenCV/Method to enable OpenEXR on OpenCV †
#multilang(ja){{
概要
OpenCV(Windows)でOpenEXRの画像読み書きを有効にする方法を紹介する.
下記のバージョンで確認を行った.
- Windows Vista 32bit
- OpenCV SVN rev6330(未確認ですがOpenCV 2.3.0では動作しないと思われます)
- Visual Studio 2010 pro
必要なファイル
OpenEXR
- OpenEXR公式サイトから下記のファイルをダウンロードする.
- ilmbase-1.0.2.tar.gz
- openexr-1.7.0.tar.gz
zlib
- zlib公式サイトから下記のファイルをダウンロードする.
サンプル画像
- OpenEXR公式サイトから下記のファイルをダウンロードする.
- openexr-images-1.7.0.tar.gz
ファイル配置
前述のファイルを展開して,下記のように配置する.
DeployディレクトリはOpenEXRのDLL,ライブラリ,ヘッダがコピー,生成されるディレクトリである.
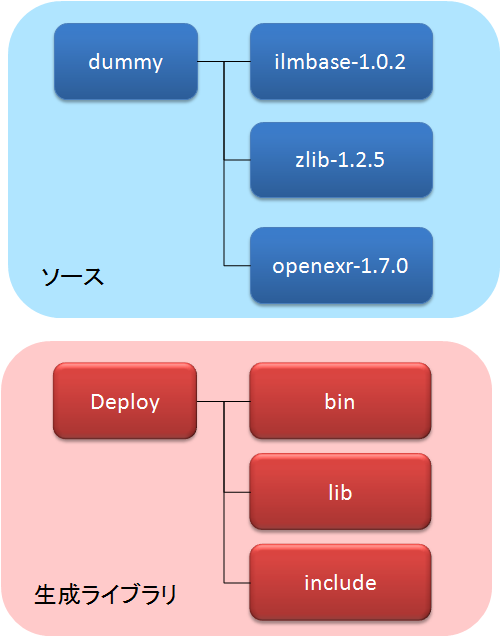
OpenEXRのビルド
Ilmbaseのビルド
- ilmbase-1.0.2.tar.gzを展開し,Visual Studioで下記のファイルを開く.
ilmbase-1.0.2.tar\ilmbase-1.0.2\vc\vc8\IlmBase.sln
- 下記の設定でソリューションをビルド.ビルド完了後,Deployディレクトリが生成される.
ソリューション構成 | Release |
ソリューションプラットフォーム | Win32 |
zlibのビルド
- zlib125.zipを展開する.
- Visual Studio 2010コマンドプロンプトでzlib-1.2.5\contrib\masmx86に移動して下記コマンドを実行する.
bld_ml32.bat
- Visual Studioで下記のファイルを開く.
zlib-1.2.5\contrib\vstudio\vc10\zlibvc.sln
- zlibvcプロジェクトを下記の構成でビルドする.
ソリューション構成 | Release |
ソリューションプラットフォーム | Win32 |
- zlib-1.2.5\contrib\vstudio\vc10\x86\ZlibDllRelease?にDLL,ライブラリが生成される.
- 下記DLLをDeploy\bin\Win32\Releaseにコピー.
zlibwapi.dll
- 下記ライブラリをDeploy\lib\Win32\Releaseにコピー.
zlibwapi.lib
zlibwapi.map
- zlib-1.2.5にある下記ヘッダファイルをDeploy\includeにコピー.
zlib.h
zconf.h
IlmImf?のビルド
- openexr-1.7.0.tar.gzを展開し,Visual Studioで下記のファイルを開く.
openexr-1.7.0\vc\vc8\OpenEXR\OpenEXR.sln
- IlmImf?プロジェクトを下記の構成でビルドする.
ソリューション構成 | Release |
ソリューションプラットフォーム | Win32 |
OpenCVのビルド
- CMakeでWITH_OPENEXRをONにする
- Deployディレクトリ中のOpenEXRのインクルードパスとライブラリを指定する
- OpenCV.slnのopencv_highguiプロジェクトがリンクするライブラリとして下記ファイルを追加する
IlmThread.lib
- OpenCV.slnのopencv_highguiプロジェクトのプリプロセッサ定義に下記の値を追加する
OPENEXR_DLL
- OpenCVを下記の構成でビルドする.
ソリューション構成 | Release |
ソリューションプラットフォーム | Win32 |
サンプルプログラム
ダウンロードしておいた下記ファイル(サンプル画像)を展開する.
- openexr-images-1.7.0.tar.gz
下記にファイル読み込みのサンプルプログラムを記載する.
#include <iostream>
#include <opencv2/core/core.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/highgui/highgui.hpp>
int main(int argc, char *argv[])
{
cv::Mat src_img = cv::imread("PrismsLenses.exr", 1);
cv::namedWindow("src", CV_WINDOW_AUTOSIZE|CV_WINDOW_FREERATIO);
cv::imshow("src", src_img);
cv::waitKey(0);
return 0;
}
Contact
間違い・御指摘等ありましたら,
までメールにてご連絡ください.
}}
#multilang(en){{
Abstract
This page describes the way to enable OpenEXR on OpenCV.
I checked in the following environment.
- Windows Vista 32bit
- OpenCV SVN rev6330(Maybe, I think that this function does not work properly on OpenCV 2.3.0)
- Visual Studio 2010 pro
Required files
OpenEXR
- Please download the following files from OpenEXR Official site.
- ilmbase-1.0.2.tar.gz
- openexr-1.7.0.tar.gz
zlib
- Please download the following file from zlib Official site.
Sample images
- Please download the following files from OpenEXR Official site.
- openexr-images-1.7.0.tar.gz
File placement
Please extract the above files and placed as follows.
The OpenEXR's files(DLLs, libraries, header files) are generated to Deploy directory..
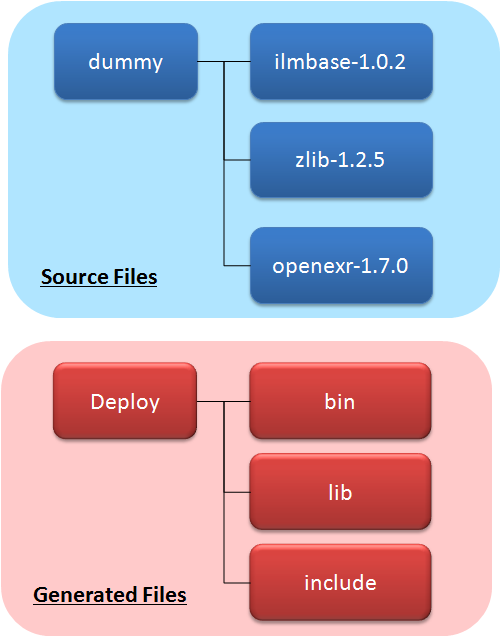
Building of OpenEXR
Building of Ilmbase
- Please extract ilmbase-1.0.2.tar.gz and open the following file in Visual Studio.
ilmbase-1.0.2.tar\ilmbase-1.0.2\vc\vc8\IlmBase.sln
- Please build the solution with the following settings. After the building, Deploy directory is created.
Solution Configuration | Release |
Solution Platform | Win32 |
Building of zlib
- Please extract zlib125.zip.
- Please launch Visual Studio 2010 Command Prompt and move to zlib-1.2.5\contrib\masmx86
- Please run the following command.
bld_ml32.bat
- Please open the following file in Visual Studio.
zlib-1.2.5\contrib\vstudio\vc10\zlibvc.sln
- Please build the zlibvc project with the following settings.
Solution Configuration | Release |
Solution Platform | Win32 |
After the building, DLLs and liraries are generated to zlib-1.2.5\contrib\vstudio\vc10\x86\ZlibDllRelease?..
- Please copy the following DLL to Deploy\bin\Win32\Release.
zlibwapi.dll
- Please copy the following libraries to Deploy\lib\Win32\Release.
zlibwapi.lib
zlibwapi.map
- Please copy the following header files in zlib-1.2.5 to Deploy\include.
zlib.h
zconf.h
Building of IlmImf?
- Please extract openexr-1.7.0.tar.gz and open the following file in Visual Studio.
openexr-1.7.0\vc\vc8\OpenEXR\OpenEXR.sln
- Please build the IlmImf? project with the following settings.
Solution Configuration | Release |
Solution Platform | Win32 |
Building of OpenCV
- Please enable WITH_OPENEXR on CMake.
- Please specify the include path and library for OpenEXR in the Deploy directory.
- Please add the following file to opencv_highgui project in OpenCV.sln as a linked library.
IlmThread.lib
- Please add the following preprocessor definition to opencv_highgui project in OpenCV.sln
OPENEXR_DLL
- Please build the OpenCV with the following settings.
Solution Configuration | Release |
Solution Platform | Win32 |
Sample program
Please extract the following zip flie.
- openexr-images-1.7.0.tar.gz
I describes a sample program to read OpenEXR image.
#include <iostream>
#include <opencv2/core/core.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/highgui/highgui.hpp>
int main(int argc, char *argv[])
{
cv::Mat src_img = cv::imread("PrismsLenses.exr", 1);
cv::namedWindow("src", CV_WINDOW_AUTOSIZE|CV_WINDOW_FREERATIO);
cv::imshow("src", src_img);
cv::waitKey(0);
return 0;
}
Contact
If you have question,please send e-mail to the following address.

}}